Hi everyone!
To celebrate Parkitects 6th anniversary, we’ve got a new free update for the game along with some exciting news to share!
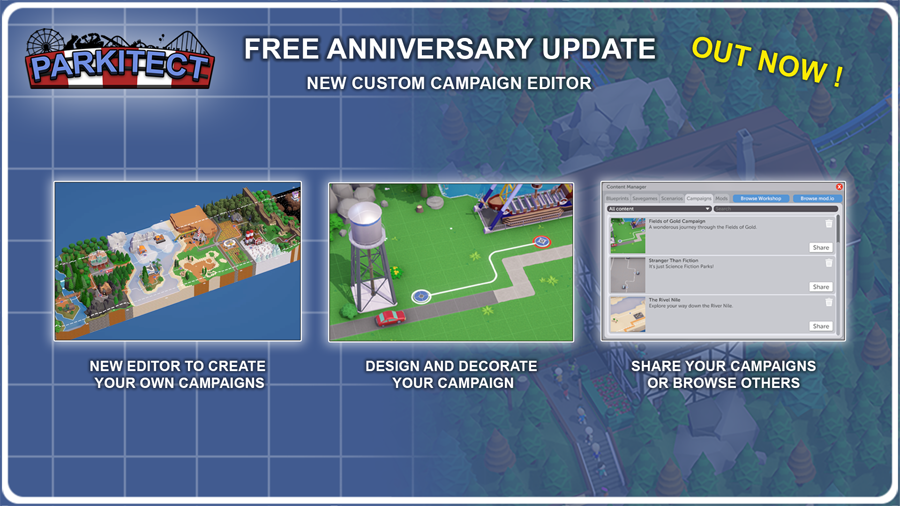
Custom Campaign Editor
This update adds a new custom campaign editor to the game in addition to the scenario editor.
Now you’ll be able to create and play your own custom campaigns, just like the ones that come with the base game and the Taste of Adventure DLC.
The campaigns can also be shared online through Steam Workshop and mod.io, so you're able to play the custom campaigns created by others.
The amazing AstroTron has already turned some of his scenarios into the brand new custom campaign that you see in the video above.
It's available on Steam Workshop here.
Big thanks to AstroTron for testing the campaign editor and allowing us to use the campaign in our showcase video!
New DLC Incoming
We’re also very excited to finally announce that a new content DLC is in the works and should be released in a few months. We know many of you have been asking for this, and we’re excited to have the opportunity to make it happen!
Work on it started fairly recently so there's nothing to show yet, but so far we know that it'll be a smaller DLC than the existing ones that'll focus on new deco themes.
We’ll be sharing more details later as development progresses.
AMA today
To top things off, we thought it would be fun to run another AMA (ask me anything) over on Reddit to mark this occasion. If you have any questions for us about Parkitect, Croakwood or game development in general, head over to Reddit and we’ll answer as best we can. The AMA starts at 6pm CET / 9am PST.
reddit AMA
A big thanks to our amazing community and our sincere thanks to all our players, for all these wonderful years! Happy anniversary everyone!